Table of Contents
Introduction
When building document processing pipelines, choosing the right OCR service is crucial. We recently implemented Mistral OCR in our startup data processing system at JobAssure, and here’s our experience comparing it with JigsawStack vOCR.
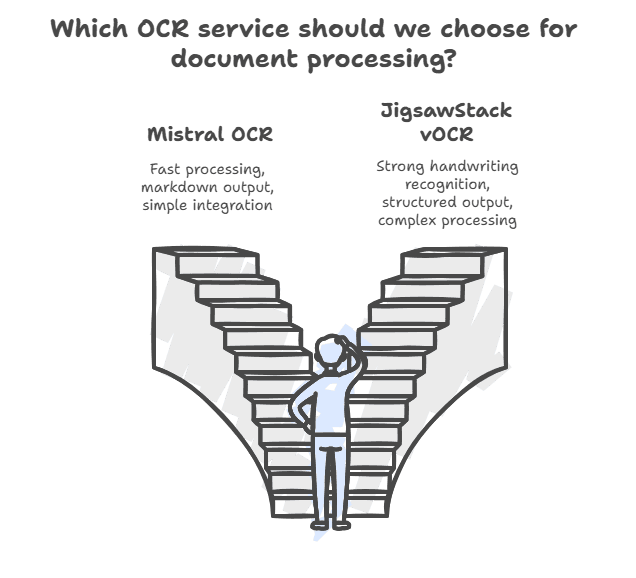
Feature Comparison
Feature | Mistral OCR | JigsawStack vOCR |
---|---|---|
Multilingual Support | Excellent | Excellent |
Handwriting Recognition | Limited | Strong |
Structured Output | Markdown/JSON | JSON/CSV |
API Response Time | 1-3s | 2-5s |
Pricing | Pay-per-use | Tiered |
Implementation Insights
Here’s how we integrated Mistral OCR in our TypeScript backend:
// Simplified version of our mistralOcr.service.ts
class OcrService {
private mistralai: Mistral;
constructor() {
this.mistralai = new Mistral({ apiKey: env.MISTRALAI_API_KEY });
}
public async processDocument(documentUrl: string): Promise<StartupData[]> {
const options = {
model: "mistral-ocr-latest",
responseFormat: "json",
document: { type: "document_url", documentUrl }
};
const result = await this.mistralai.ocr.process(options);
return this.parseMarkdownTables(result.pages);
}
}
Advanced Features Deep Dive
Mistral OCR’s Strengths
- Markdown Parsing Magic
- Automatic table detection with markdown formatting
- Preserves document hierarchy (headings, lists)
- Example from our implementation:
// In mistralOcr.service.ts
private parseMarkdownTable = (OcrPage: OCRPageObject): IStartupDetailsDTO[] => {
const markdown = OcrPage.markdown;
const lines = markdown.split('\n').map(line => line.trim()).filter(line => line);
// Advanced parsing logic for financial data
// ...
}
- Batch Processing
- Can process 50+ page documents in single API call
- Maintains document structure across pages
JigsawStack vOCR’s Advanced Capabilities
-
Document Intelligence
- Entity extraction (dates, amounts, names)
- Document classification (invoice vs receipt)
- Custom field extraction templates
-
Post-Processing Pipeline
- Built-in data validation
- Automatic data normalization
- Confidence scoring per field
Real-World Use Cases
Startup Funding Analysis (Our Implementation)
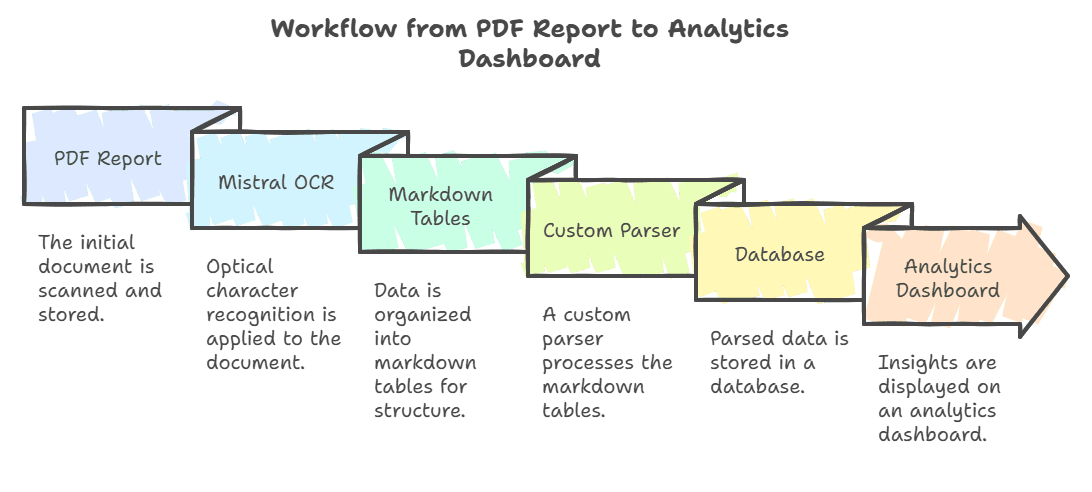
Why we chose Mistral:
- Needed raw markdown for custom financial data parsing
- Fast processing of VC funding reports (50+ pages)
- Simple integration with our TypeScript backend
Alternative Use Cases for JigsawStack
-
Medical Forms Processing
- Handwritten patient intake forms
- Structured output for EHR integration
- HIPAA-compliant processing
-
Legal Document Analysis
- Contract clause extraction
- Signature detection
- Redaction capabilities
Mock Data Processing
Let’s see how both services handle different document types:
1. Financial Report (PDF)
| **Quarter** | **Revenue** | **Profit** |
|-------------|-------------|------------|
| Q1 | $1.2M | $200K |
| Q2 | $1.5M | $300K |
Mistral Output:
{
"pages": [{
"markdown": "| Quarter | Revenue | Profit |...",
"confidence": 0.97
}]
}
JigsawStack Output:
{
"tables": [{
"headers": ["Quarter", "Revenue", "Profit"],
"rows": [["Q1", "1.2M", "200K"]]
}]
}
Performance Benchmarks
Metric | Mistral OCR | JigsawStack |
---|---|---|
10-page PDF | 1.2s | 2.8s |
Handwriting | 65% acc. | 89% acc. |
Table Detection | 98% acc. | 92% acc. |
API Limits | 1000/min | 500/min |
Integration Tips
- Error Handling
try {
const data = await ocrService.processDocument(url);
} catch (error) {
// Mistral-specific error handling
if (error.response?.status === 429) {
// Implement retry logic
}
}
- Webhook Support
- Both services offer webhook for async processing
- JigsawStack provides more detailed status updates
Future Considerations
- Mistral’s Roadmap: Better handwriting support in Q3 2025
- JigsawStack: Custom model training coming soon
Recommendations
Based on our implementation:
-
Choose Mistral if you need:
- Fast processing of clean documents
- Markdown output for easy parsing
- Simple API integration
-
Choose JigsawStack if you need:
- Better handwriting recognition
- More structured output formats
- Complex document processing